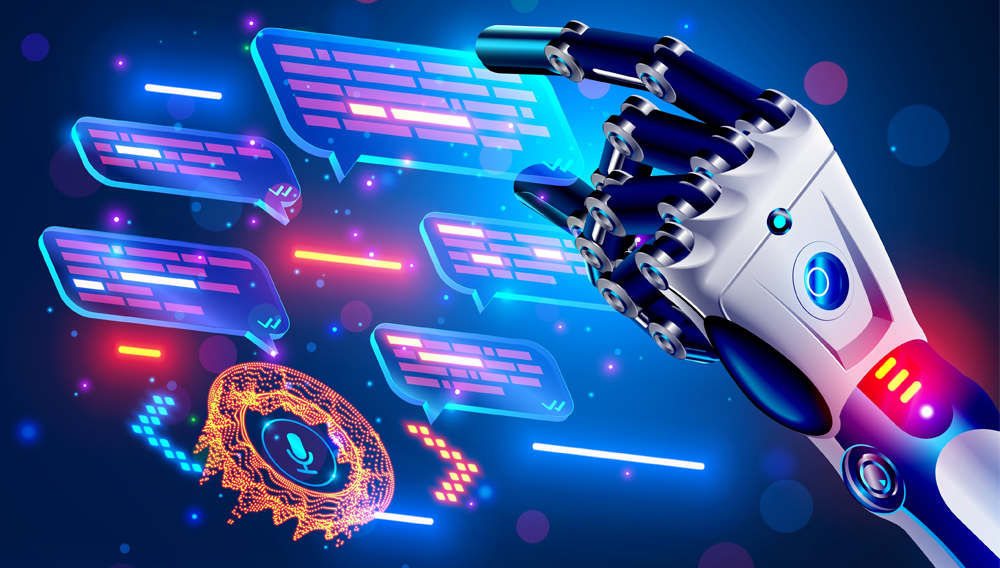
4 min read
Introducing Connext AI: An Entirely New Way to Develop Smart Physical Systems
I’ve been waiting a long time. And now it’s here. This week.
Read more ⇢RTI is the world’s largest DDS supplier and Connext is the most trusted software framework for critical systems.
|
From downloads to Hello World, we've got you covered. Find all of the tutorials, documentation, peer conversations and inspiration you need to get started using Connext today.
RTI provides a broad range of technical and high-level resources designed to assist in understanding industry applications, the RTI Connext product line and its underlying data-centric technology.
The monthly RTI Newsletter lets you in on what’s happening across all the industries that matter to RTI customers.
RTI is the software framework company for physical AI systems. RTI Connext delivers the reliability, security and performance essential for highly distributed autonomous and physical AI systems.
Your Systems. Working as One.
4 min read
I’ve been waiting a long time. And now it’s here. This week.
Read more ⇢4 min read
In many major U.S. cities on the East and West coast, urban gridlock is a significant obstacle that slows commerce, negatively affects the...
2 min read
Mission-critical systems, such as autonomous vehicles and robotic-assisted surgery, cannot afford to fail. They must operate flawlessly under any...
3 min read
In today's increasingly containerized world, understanding how to efficiently monitor and manage your Docker containers running RTI Connext...
3 min read
In any field, whether it's sports, business, or personal development, taking the time to train before starting an important high-stakes endeavor can...
4 min read
In my discussions with traditional OEM automotive executives, three topics consistently emerge as primary concerns: software-defined vehicles, AI,...
2 min read
Tired of sifting through irrelevant search engine results to find answers for your Connext projects? The Connext Chatbot is here to help!
4 min read
The concept of the OODA Loop – Observe, Orient, Decide, and Act – was developed by military strategist John Boyd. It is focused on the ability to...
4 min read
In today's rapidly evolving global landscape, the defense sector is experiencing a transformation marked by several critical trends. These...
4 min read
In the rapidly evolving landscape of medical technology, surgical robots are becoming increasingly sophisticated and interconnected. With this...
Connext is the world's leading implementation of the Data Distribution Service (DDS) standard for Real-Time Systems. Try a fully-functional version of Connext for free.